In today’s digital landscape, understanding user behavior is essential for enhancing user experience and improving website performance. One important aspect of this is knowing when users leave a page on your website. Whether you’re aiming to reduce bounce rates, optimize your content, or enhance your web applications, being able to track user exits can provide invaluable insights. In this article, we will explore how to achieve this in PHP, covering various methods, their implementation, and best practices.
Why Tracking User Exits Matters
Before diving into the technicalities of user exit tracking in PHP, it’s important to establish why this practice is beneficial. Here are some reasons:
- Improved User Experience: By understanding when and why users leave, you can adjust your content or design to keep them engaged longer.
- Increased Conversion Rates: Knowing where users drop off can help you optimize critical areas of your website that lead to conversions.
Understanding user behavior paves the way for better decision-making and ultimately enhances the effectiveness of your website or application.
Methods to Detect User Exits in PHP
Detecting when a user leaves a page requires a combination of client-side and server-side techniques. PHP operates on the server side, but it can work hand-in-hand with JavaScript, which runs on the client side. Below, we’ll explore the main techniques for tracking user exits:
1. Using JavaScript Events
JavaScript provides various events that can help detect user exits. The most relevant ones for our purpose are the beforeunload
and unload
events.
Implementing the `beforeunload` Event
The beforeunload
event allows you to execute JavaScript code just before the user leaves the page. Here’s how you can leverage this event with PHP:
“`html
“`
In this snippet, we use navigator.sendBeacon
to send a request to a PHP script (exit_tracker.php
) whenever the user is about to leave. This is particularly useful because sendBeacon
doesn’t block the unloading of the document, ensuring the data is sent without slowing down the exit process.
Processing the Data in PHP
The exit_tracker.php
script will handle incoming requests. Here’s a simple implementation:
“`php
“`
In this PHP code, we’re reading the JSON data sent from the JavaScript and logging the user’s exit along with the timestamp in a text file called exit_log.txt
.
2. Tracking Mouse Events with JavaScript
Another method is to track mouse movements. If the mouse leaves the viewport or the user moves it towards the top edge of the browser, there’s a high chance they might exit the page.
Detecting Mouse Leave
You can set up an event listener for the mouseleave
event. Here’s how this can be done:
“`html
```
This script sends a request to your exit_tracker.php
when the mouse leaves the viewport, particularly targeting the upper part of the browser window.
Advantages and Disadvantages of Client-Side Tracking
While client-side tracking is effective, it also has its drawbacks. Here are some of the advantages and disadvantages:
Advantages
- Real-Time Insights: You can get immediate feedback on user interactions and exits as they occur.
- Less Server Load: Client-side scripts can reduce the number of requests sent to the server compared to extensive server-side checks.
Disadvantages
- Dependence on JavaScript: If users have JavaScript disabled, this method won't work.
- Limited Data Accuracy: Users can navigate away quickly before the exit tracking code runs.
Combining PHP with Other Methods for Comprehensive Tracking
To gain a full understanding of user behavior, it's beneficial to combine PHP with other analytics tools alongside your custom implementation.
Integrating Google Analytics
Google Analytics provides robust tracking capabilities. You can set up Event Tracking to see how often users leave a page. Here's how:
- Set up a new Event in Google Analytics for user exits.
- Use the same JavaScript techniques above to send an event when the user is about to leave.
For example:
```html
```
This event can then be tracked in your Google Analytics dashboard to capture user exit data.
Analyzing User Behavior with PHP
In addition to exit tracking, PHP can be utilized to analyze user behavior once they’ve exited. By processing log files or using a database, you can evaluate patterns and optimize your strategies accordingly.
Implementing a Robust Exit Tracking System
To create a sophisticated exit tracking system, you will need to consider the following:
1. Data Storage
Logging exits to a text file may not be sufficient for large-scale applications. Consider utilizing a database (such as MySQL) to store exit data. Here is a simple table structure:
sql
CREATE TABLE user_exits (
id INT AUTO_INCREMENT PRIMARY KEY,
page VARCHAR(255) NOT NULL,
reason VARCHAR(50),
exit_time DATETIME DEFAULT CURRENT_TIMESTAMP
);
In your PHP exit tracking script, you can then insert a new record into this table.
2. Data Analysis
Once you have your data organized, utilize PHP to analyze it and comprehend user exit patterns. For example, you can create reports showing:
- Which pages have the highest exit rates.
- The average duration users spent on each page before exiting.
Leveraging these metrics can guide your optimization efforts.
Best Practices for Tracking User Exits
To ensure comprehensive and ethical tracking of user exits, consider implementing these best practices:
- Privacy Compliance: Always inform users about tracking and ensure compliance with regulations like GDPR.
- Performance: Ensure that the methods employed do not degrade user experience. Use asynchronous requests where possible.
Conclusion
Tracking when a user leaves a page can significantly impact your understanding of user behavior and website performance. By utilizing a combination of JavaScript events and PHP back-end processing, you can effectively gather exit data to inform your strategies and enhance user experience. Whether it’s for improving conversion rates or refining your content, an effective exit tracking method is essential for any modern web application.
By implementing the techniques and practices discussed in this article, you'll be well on your way to mastering user exit tracking in PHP, paving the way for a more data-driven approach to enhancing your website.
What is user exit tracking in PHP?
User exit tracking in PHP refers to the practice of monitoring when users leave a webpage or site. This can involve tracking various events that indicate a user is about to navigate away, such as moving the mouse to close the tab, clicking on a link to another site, or simply scrolling away from the current page. By implementing user exit tracking, website owners and developers can gather valuable insights into user behavior and engagement.
This data can be beneficial for understanding why users leave your site, identifying content that may need improvement, or recognizing key areas that keep users engaged. It can also help with analytics by providing metrics about user retention and exit rates, which are crucial for enhancing user experience and site performance.
How does user exit tracking work in PHP?
User exit tracking generally works through JavaScript, which observes user interactions on the webpage. Events such as mouseleave
, beforeunload
, or specific link clicks can trigger a tracking function that sends data to a PHP script on the server. This script then logs the information into a database or an analytics platform for further analysis.
When a user is about to leave the site, the JavaScript can execute a function that collects relevant data, such as the URL of the page, the time spent on the page, and potentially some user-specific information. This data is then sent to the server-side PHP script via AJAX, allowing for real-time tracking and storage of user actions.
What are the benefits of implementing user exit tracking?
Implementing user exit tracking provides multiple benefits for website owners. Firstly, it helps to understand the user journey by analyzing the points at which users tend to leave. This analysis can reveal patterns that may indicate potential issues with content or site navigation that need improvement. Moreover, it also aids in assessing the effectiveness of different pages or content types in retaining visitors.
Secondly, user exit tracking can enhance marketing efforts by providing insights into user behavior. Knowing when and why users leave can inform strategies for optimization and engagement, such as creating targeted campaigns or improving call-to-action placements. Ultimately, this data can drive decisions that aim to enhance user retention and conversion rates on the website.
What are the technical requirements for user exit tracking in PHP?
To implement user exit tracking in PHP, a basic knowledge of both PHP and JavaScript is essential. You will need the ability to write JavaScript code that can handle events such as mouseleave
or beforeunload
. Additionally, you will need to create a PHP script that can process incoming data and store it in a database securely.
On the technical side, ensure your server can handle AJAX requests and that you have a database set up for storing exit tracking data. Familiarity with libraries like jQuery can also simplify event handling in JavaScript. Proper implementation involves both client-side scripting to detect exits and server-side scripting to record user data effectively.
Can user exit tracking impact website performance?
User exit tracking can have an impact on website performance, but it is generally minimal if implemented correctly. Adding additional JavaScript to monitor exits can slightly increase loading times, especially if there are multiple tracking scripts present. However, if optimized properly, the impact can be negligible.
To ensure performance remains high, developers should keep scripts lightweight and efficient, minimizing the number of resources loaded on page exit events. Furthermore, asynchronous data submission methods, like AJAX, can help reduce any potential delays in user experience while still capturing necessary exit tracking data.
Is it ethical to track user exits on my website?
Yes, tracking user exits can be ethical as long as it is done transparently and in compliance with privacy regulations. Users should typically be informed that their interactions are being tracked, either through a privacy policy or a cookie consent banner. Consent is key, and ensuring users are aware of tracking practices fosters trust.
It's also important to anonymize the data collected to protect user privacy. Avoid collecting personal information unless absolutely necessary, and only retain data for a limited time according to your privacy policy. By prioritizing user consent and data protection, you can ethically implement user exit tracking on your website.
What tools can be used for user exit tracking?
Several tools and libraries can be utilized for user exit tracking, with a combination of PHP and JavaScript being the most common approach. Google Analytics, for instance, can be extended to capture exit events using custom events and triggers, simplifying the process for those already utilizing the platform.
Additionally, libraries like jQuery can be used to help capture mouse movement or clicks efficiently. Other specialized tools, such as Hotjar or Crazy Egg, provide built-in functionalities for tracking user behavior, including exit tracking. These tools offer analytical capabilities that require less development work compared to building a custom solution from scratch.
How can I analyze the data collected from user exit tracking?
Analyzing data collected from user exit tracking can be done using various data analysis tools or by integrating with platforms like Google Analytics. Once the data is stored in a database, you can utilize PHP to query that data and generate reports. The data can include metrics like exit rates, pages with the highest exits, and user behavior leading up to the exit.
Using visualization tools, such as Google Data Studio or Tableau, can help present the data in an easily interpretable format. By analyzing trends and patterns, you can identify problem areas on your site, inform design and content decisions, and ultimately create a better user experience that encourages visitors to stay longer.
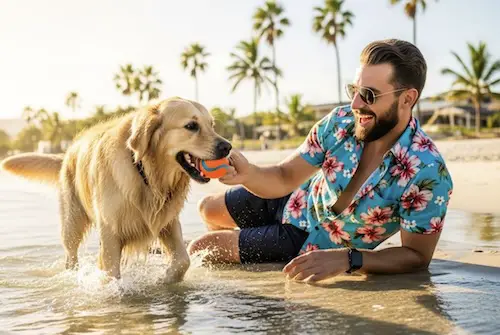
I’m passionate about making home cooking simple, enjoyable, and stress-free. Through years of hands-on experience, I share practical tips, smart meal prep ideas, and trusted kitchen essentials to help you feel more confident in the kitchen every day.